Day 5 : More Advanced Circuits
In order to continue this course, please have a Raspberry Pi Pico and electronic components available:
Raspberry Pi Pico: Raspberry Pi Pico Amazon Link
Electronic Components: Electronic Components Amazon Link
Day 5 Quiz: Please take in order to gauge your understanding of Day 4 concepts
Getting the Temperature
Building a circuit to determine the temperature
Import necessary modules:
-
machine: This module provides access to hardware components and pins on the microcontroller.
-
time: This module is used for time-related functions
Initialize the temperature sensor:
-
sensor_temp = machine.ADC(4): This line initializes an Analog-to-Digital Converter (ADC) object on pin 4, which is likely connected to a temperature sensor
Calculate the conversion factor:
-
conversion_factor = 3.3 / 65535: This line calculates a conversion factor to convert the ADC readings (ranging from 0 to 65535) into a voltage range of 0-3.3 volts.
Start an infinite loop:
-
while True:: This creates an infinite loop, so the code keeps running until it is manually interrupted or stopped.
-
Inside the loop:
-
Read the temperature sensor:
-
reading = sensor_temp.read_u16(): This line reads the analog value from the temperature sensor and stores it as reading. read_u16() reads a 16-bit unsigned integer value from the ADC.
-
Convert the ADC reading to temperature:
-
temperature = 27 - (reading - 0.706) / 0.001721: This line calculates the temperature in degrees Celsius using a formula specific to the temperature sensor. It assumes a linear relationship between the ADC readings and temperature, and you may need to adjust these coefficients based on the sensor's datasheet. The calculated temperature is stored in the variable temperature.
-
Print the temperature:
-
print(temperature): This line prints the calculated temperature to the console.
-
Pause for 2 seconds:
-
utime.sleep(2): This line pauses the loop for 2 seconds before starting the next iteration, providing a delay between temperature readings.
Figure 23

In summary, this code reads data from an analog temperature sensor, converts it into a temperature value, and continuously prints the temperature in degrees Celsius with a 2-second delay between readings. The exact formula used to convert the ADC readings to temperature will depend on the specific temperature sensor in use.
LED Bar Display
Components Needed:
- Raspberry Pi Pico
- LED Bar
- Breadboard
- Jumper Wires
- Resistors
Steps:
1. Placement of Components:
-
Place the Raspberry Pi Pico on one side of the breadboard.
-
Position the LED bar display in the middle of the breadboard, between the two sets of rows.
2. Connecting the GPIO Pin:
-
Use a jumper wire to connect a GPIO pin of the Raspberry Pi Pico to the positive rail of the breadboard. In your diagram, this is GPIO pin 2.
3. Resistors and LED Bar Connection:
-
Insert resistors into the breadboard. Connect one end of each resistor to the positive rail.
-
Connect the other end of each resistor to the corresponding pin (or metal peg) of the LED bar display. This step limits the current to each LED, preventing damage.
4. Connecting to the Negative Rail:
-
Use additional jumper wires to create connections from the LED bar display to the negative rail of the breadboard. Each LED in the bar display should be connected to the negative rail through a separate jumper wire.
5. Completing the Circuit:
-
Finally, connect a jumper wire from the negative rail on the breadboard to a ground (GND) pin on the Raspberry Pi Pico. This step completes the circuit.
Figure 24
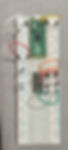
Great Job! Now let's add code to have the LED bar light up!
10. Add the following lines that
can be seen in Figure 25
11. This will cause provide power towards GPIO Pin 2. Since the jumper wrie is connected to GPIO Pin 2, and the resistors are conected to that wire, all of the conncted LED Strips will turn on!
Figure 25

RGB LED
Components Needed:
-
Raspberry Pi Pico
-
Breadboard
-
3 LEDs (blue, green, red)
-
3 resistors (220-1k ohm)
-
Jumper wires
Steps:
1. Place the LEDs on the Breadboard:
-
Insert the blue LED into row 38, ensuring the longer leg (anode) is in one column and the shorter leg (cathode) in an adjacent column.
-
Insert the green LED into row 32 in a similar manner.
-
Insert the red LED into row 27, following the same approach
2. Connect Resistors to GPIO Pins:
-
Connect one end of a resistor to GPIO pin 13 on the Raspberry Pi Pico and the other end to the column where the anode of the blue LED is placed.
-
Connect another resistor from GPIO pin 14 to the anode column of the green LED.
-
Connect the last resistor from GPIO pin 15 to the anode column of the red LED.
3. Complete the LED Circuits:
-
Use jumper wires to connect the cathode columns of each LED to the negative (ground) rail on the breadboard.
-
Ensure each LED is connected to the ground rail via its own jumper wire.
4. Connect the Ground Rail to Raspberry Pi Pico:
-
Use another jumper wire to connect the negative rail on the breadboard to one of the ground (GND) pins on the Raspberry Pi Pico. This completes the ground connection for all LEDs.
Figure 26
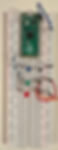
Great Job! Now let's add our code to have our LED blink at different intervals!
10. Add the following lines that
can be seen in Figure 27
11. This will cause your LED lights
to turn on and off in 1 second
intervals
Figure 27

LED Potentiometer Circuit
Components Needed:
-
Raspberry Pi Pico: The microcontroller board that will control the LEDs.
-
LEDs:
-
1 Blue LED
-
1 Green LED
-
1 Red LED
-
-
3 Potentiometers
-
3 Resistors (220-ohm to 1K-ohm)
-
10 Jumper Wires
-
Breadboard
1. Place the LEDs and Potentiometers on the Breadboard:
-
Blue LED: Insert the blue LED in row 38.
-
Green LED: Insert the green LED in row 27.
-
Red LED: Insert the red LED in row 12.
-
Potentiometers: Insert three potentiometers on the breadboard. Ensure the leftmost metal leg (typically the one controlling the resistance) of each potentiometer is in the same row as its corresponding LED.
2. Connect the Raspberry Pi Pico to the Breadboard:
-
Use a jumper wire to connect GPIO pin 10 on the Raspberry Pi Pico to the negative rail on the left side of the breadboard.
-
Connect another jumper wire from GPIO pin 11 to the positive rail on the breadboard.
3. Wire the LEDs to the Raspberry Pi Pico:
-
Green LED: Connect a resistor from the negative rail to the row containing the green LED.
-
Red LED: Connect a resistor from the positive rail to the row containing the red LED.
-
Blue LED: Connect a resistor from GPIO pin 15 to the row containing the blue LED.
4. Wire the Potentiometers:
-
For each potentiometer, connect a jumper wire from the row containing the rightmost metal leg (usually the output) to the positive rail on the breadboard.
5. Complete the Circuit:
-
For each LED, connect a jumper wire from its row to the negative rail on the breadboard.
-
Connect a jumper wire from the negative rail on the breadboard to a ground (GND) pin on the Raspberry Pi Pico.
-
Finally, connect a jumper wire from the positive rail on the breadboard to the 3V3 (3.3V) pin on the Raspberry Pi Pico.
Steps:
Figure 28
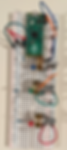
Great Job! Now let's add a bit more to our code to have our LED stay on by the button!
10. Add the following lines that
can be seen in Figure 29
11. This will let your LED circuit become
more interactive!
Figure 29

Challenges
Challenge 1: Create a circuit that makes three LED blink on and off at the same time using a GPIO pin on the Raspberry Pi Pico
Challenge 2: Create a circuit where an buzzer turns on/off based on the state of a push button. (make sure to use a resister)
Challenge 3: Build a circuit that uses a potentiometer to control the brightness of an LED bar graph.
The answers for these challenges, will not be provided as we really want you to figure it out on your own. If you are still having trouble after many attempts please contact us at groundupcs@gmail.com and we will help!